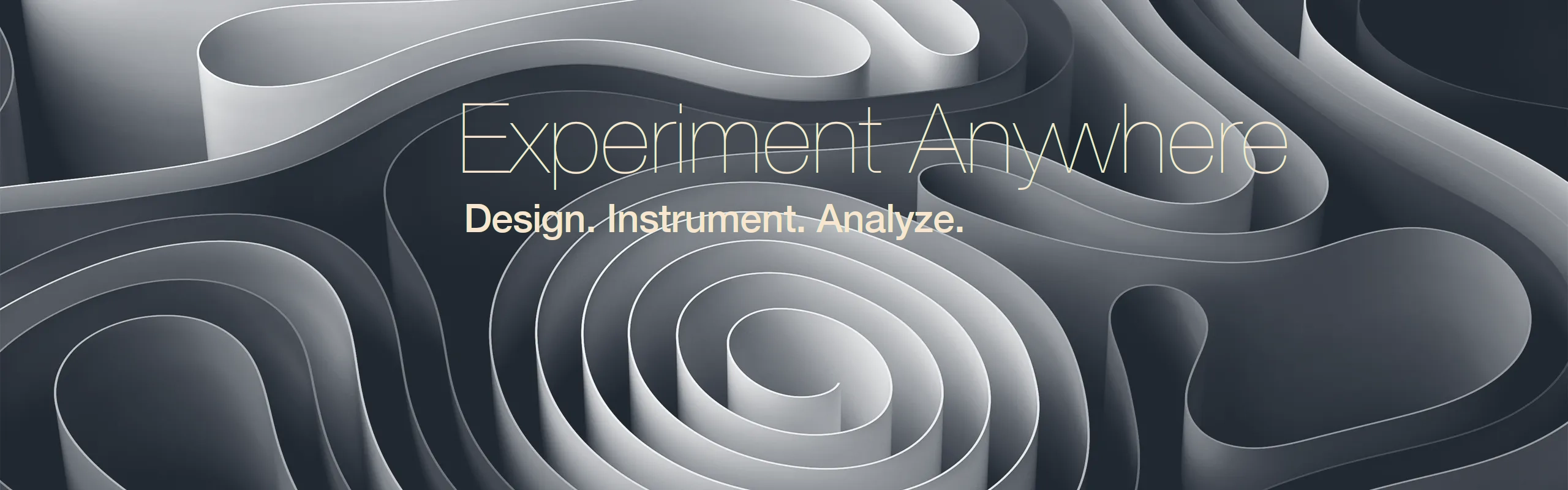
Infinitely scalable testing and feature flagging for the enterprise.
On-prem or managed PaaS.
A recent survey found that enterprises are three times as likely to abandon experimentation due to challenges instrumenting experiments as due to the complexity of statistical analysis. It should come as no surprise. The complexity of instrumentation grows dramatically (quadratically to be exact) with the number of concurrent experiments, and no off-the-shelf experimentation solution helps with instrumentation. Variant does.
Experiment everywhere
Instrument backend API, Node JavaScript, browser JavaScript, mobile client, IVR or any other interactive program. Furthermore, Variant enables cross-domain experiments spanning multiple applications.
Real time targeting and qualification
With variant you never have to worry about the vexing problems associated with the traditional pre-targeting, such as sample ratio mismatch. We only target those users who actually showed up. We also distinguish between targeting and qualification whose time-to-live can be set independently.
Instrumentation separate from implementation
The host application does not worry about the details of how new code paths are instrumented as experiments. It simply asks Variant server what code path to execute. All the complexity of generating that answer is handled by the Variant server.
Distributed session management
Variant maintains its own user sessions, which is particularly suitable for modern distributed backends. Any component of a distributed host application has access to the Variant session bound to the foreground actor.
Better data governance
No data, sensitive or not, ever leaves your warehouse. Trust no one. Not even us.
Zero overhead
All the overhead associated with computing and managing qualification and targeting decisions, experiment lifecycle, and resource provisioning is handled by the Variant server, leaving the host application unaffected regardless of the number of active experiments.
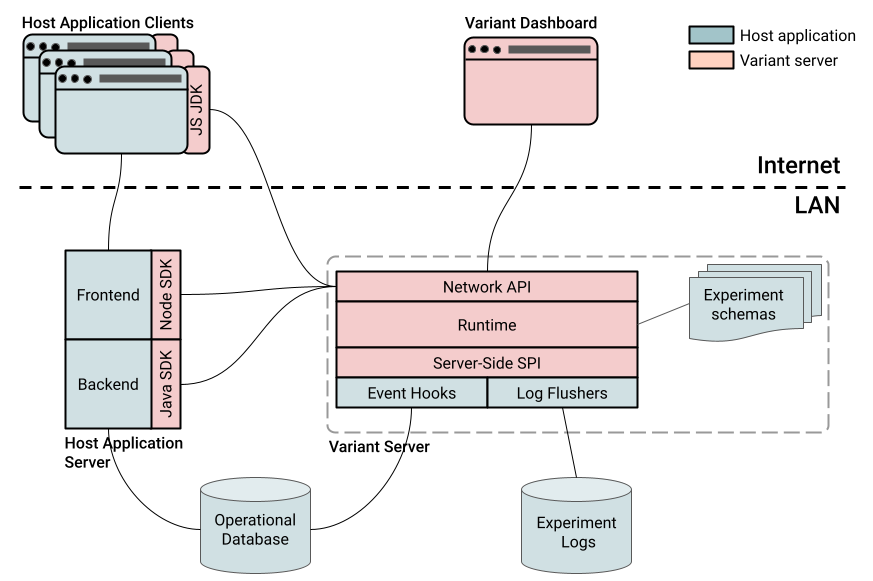